Lesson 15
Let's make a game
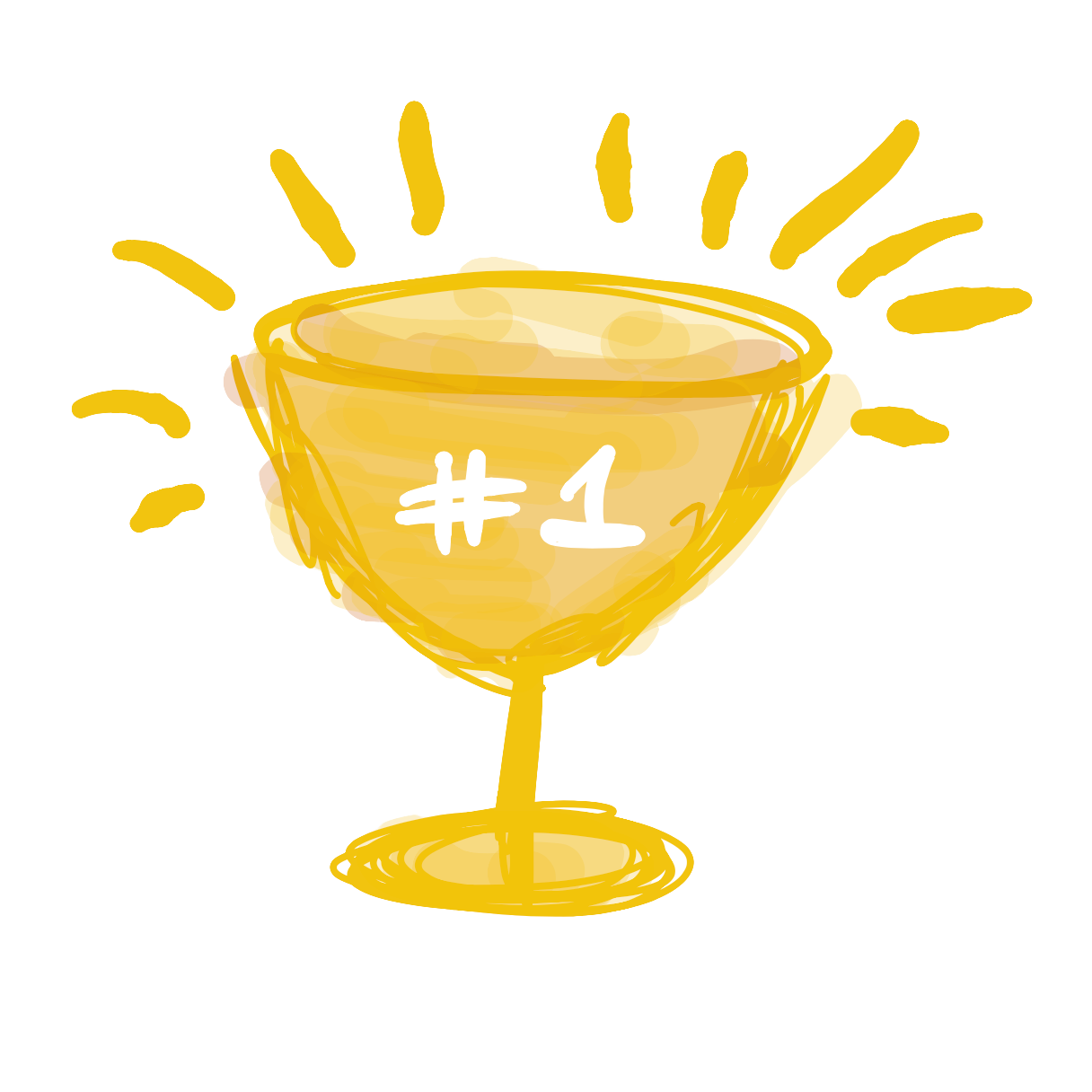
Today we are going to learn how to make this game:
Making a circle
void setup() { ellipse(50, 50, 50, 50); } void draw() { }
Now press the Play button. You should see this on your screen:
Making the canvas bigger
void setup() { size(640, 360); ellipse(50, 50, 50, 50); } void draw() { }
Now press the Play button. You should see this on your screen:
Making more circles
void setup() { size(640, 360); ellipse(50, 50, 50, 50); ellipse(10, 10, 30, 30); ellipse(100, 100, 200, 200); } void draw() { }
Now press the Play button. You should see this on your screen:
Color
void setup() { size(640, 360); ellipse(50, 50, 50, 50); fill(200, 120, 250); ellipse(10, 10, 30, 30); fill(255, 200, 200); ellipse(100, 100, 200, 200); } void draw() { }
Now press the Play button. You should see this on your screen:
Moving circle with mouse
void setup() { size(640, 360); } void draw() { fill(0,100,0); ellipse(mouseX, mouseY, 50, 50); }
Now press the Play button. You should see this on your screen:
Erasing the screen each time
void setup() { size(640, 360); } void draw() { background(255); fill(0,100,0); ellipse(mouseX, mouseY, 50, 50); }
Now press the Play button. You should see this on your screen:
Reacting to mouse press
void setup() { size(640, 360); } void draw() { background(255); ellipse(mouseX, mouseY, 50, 50); } void mousePressed() { fill(100,0,0); } void mouseReleased() { fill(0,100,0); }
Now press the Play button. You should see this on your screen:
Counting number of clicks
int clicks = 0; void setup() { size(640, 360); } void draw() { background(255); ellipse(mouseX, mouseY, 50, 50); text("Clicks: " + clicks, 100, 100, 100, 100); } void mousePressed() { clicks += 1; fill(100,0,0); } void mouseReleased() { fill(0,100,0); }
Now press the Play button. You should see this on your screen:
Adding a target
int clicks = 0; int targetX = 100; int targetY = 200; int targetSize = 50; void setup() { size(640, 360); } void draw() { background(255); ellipse(mouseX, mouseY, 50); text("Clicks: " + clicks, 100, 100, 100, 100); ellipse(targetX, targetY, targetSize); } void mousePressed() { clicks += 1; fill(100,0,0); } void mouseReleased() { fill(0,100,0); }
Now press the Play button. You should see this on your screen:
Checking if we clicked target
int clicks = 0; int targetX = 100; int targetY = 200; int targetSize = 50; void setup() { size(640, 360); } void draw() { background(255); ellipse(mouseX, mouseY, 50, 50); text("Clicks: " + clicks, 100, 100, 100, 100); ellipse(targetX, targetY, targetSize, targetSize); } void mousePressed() { int targetRadius = targetSize / 2; int targetLeft = targetX - targetRadius; int targetRight = targetX + targetRadius; int targetTop = targetY - targetRadius; int targetBottom = targetY + targetRadius; if(mouseX > targetLeft && mouseX < targetRight && mouseY > targetTop && mouseY < targetBottom) { clicks += 1; } fill(100,0,0); } void mouseReleased() { fill(0,100,0); }
Now press the Play button. You should see this on your screen:
Moving target when clicked
int clicks = 0; int targetX = 100; int targetY = 200; int targetSize = 50; void setup() { size(640, 360); } void draw() { background(255); ellipse(mouseX, mouseY, 50, 50); text("Clicks: " + clicks, 100, 100, 100, 100); ellipse(targetX, targetY, targetSize, targetSize); } void mousePressed() { int targetRadius = targetSize / 2; int targetLeft = targetX - targetRadius; int targetRight = targetX + targetRadius; int targetTop = targetY - targetRadius; int targetBottom = targetY + targetRadius; if(mouseX > targetLeft && mouseX < targetRight && mouseY > targetTop && mouseY < targetBottom) { clicks += 1; targetX = int(random(600)); targetY = int(random(300)); } fill(100,0,0); } void mouseReleased() { fill(0,100,0); }
Now press the Play button. You should see this on your screen:
Adding a timer
int clicks = 0; int targetX = 100; int targetY = 200; int targetSize = 50; int time = 100; void setup() { size(640, 360); } void draw() { background(255); ellipse(mouseX, mouseY, 50, 50); text("Clicks: " + clicks, 100, 100, 100, 100); text("Time: " + time, 100, 130, 100, 100); time -= 1; if(time <= 0) { time = 100; clicks = 0; } ellipse(targetX, targetY, targetSize, targetSize); } void mousePressed() { int targetRadius = targetSize / 2; int targetLeft = targetX - targetRadius; int targetRight = targetX + targetRadius; int targetTop = targetY - targetRadius; int targetBottom = targetY + targetRadius; if(mouseX > targetLeft && mouseX < targetRight && mouseY > targetTop && mouseY < targetBottom) { clicks += 1; time = 100; targetX = int(random(600)); targetY = int(random(300)); } fill(100,0,0); } void mouseReleased() { fill(0,100,0); }
Now press the Play button. You should see this on your screen:
Adding a high score
int clicks = 0; int highScore = 0; int targetX = 100; int targetY = 200; int targetSize = 50; int time = 100; void setup() { size(640, 360); } void draw() { background(255); ellipse(mouseX, mouseY, 50, 50); text("High Score: " + highScore, 100, 70, 100, 100); text("Clicks: " + clicks, 100, 100, 100, 100); text("Time: " + time, 100, 130, 100, 100); time -= 1; if(time <= 0) { time = 100; clicks = 0; } ellipse(targetX, targetY, targetSize, targetSize); } void mousePressed() { int targetRadius = targetSize / 2; int targetLeft = targetX - targetRadius; int targetRight = targetX + targetRadius; int targetTop = targetY - targetRadius; int targetBottom = targetY + targetRadius; if(mouseX > targetLeft && mouseX < targetRight && mouseY > targetTop && mouseY < targetBottom) { clicks += 1; if(clicks > highScore) { highScore = clicks; } time = 100; targetX = int(random(600)); targetY = int(random(300)); } fill(100,0,0); } void mouseReleased() { fill(0,100,0); }
Now press the Play button. You should see this on your screen: