Lesson 1
What is a Program?
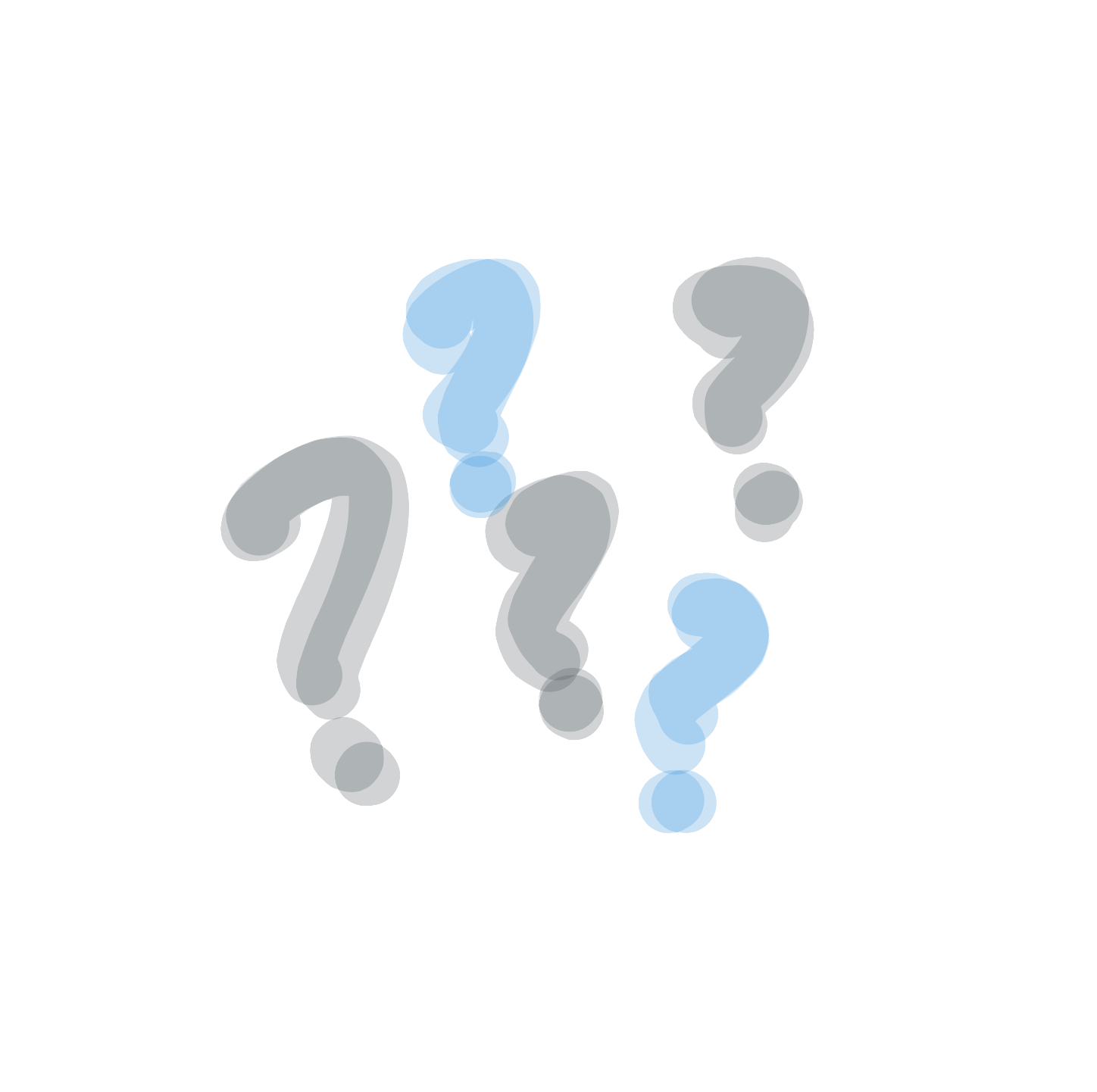
Lesson 2
Opening Processing
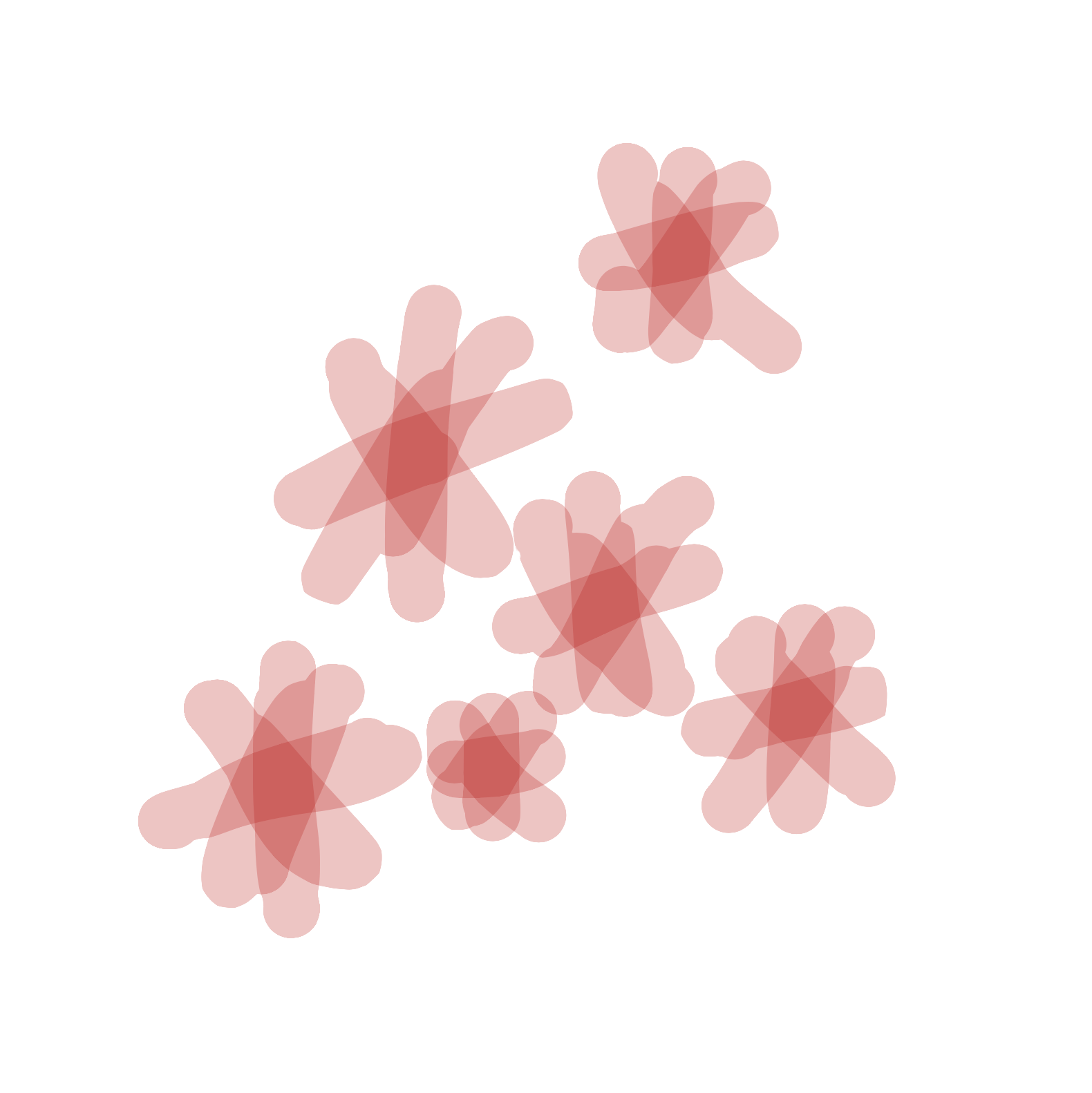
Lesson 3
Writing our first program
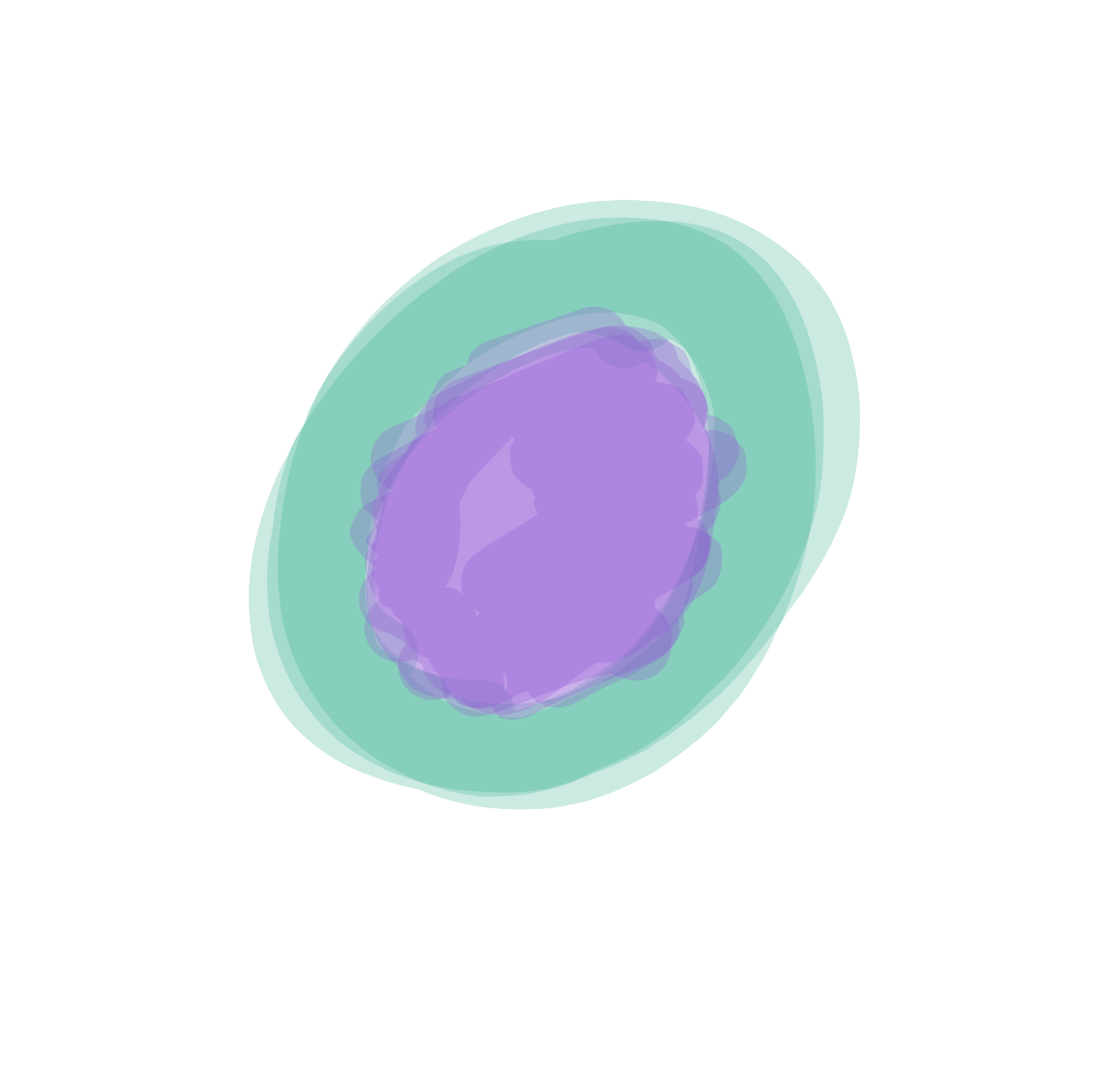
Lesson 4
Parts of an instruction
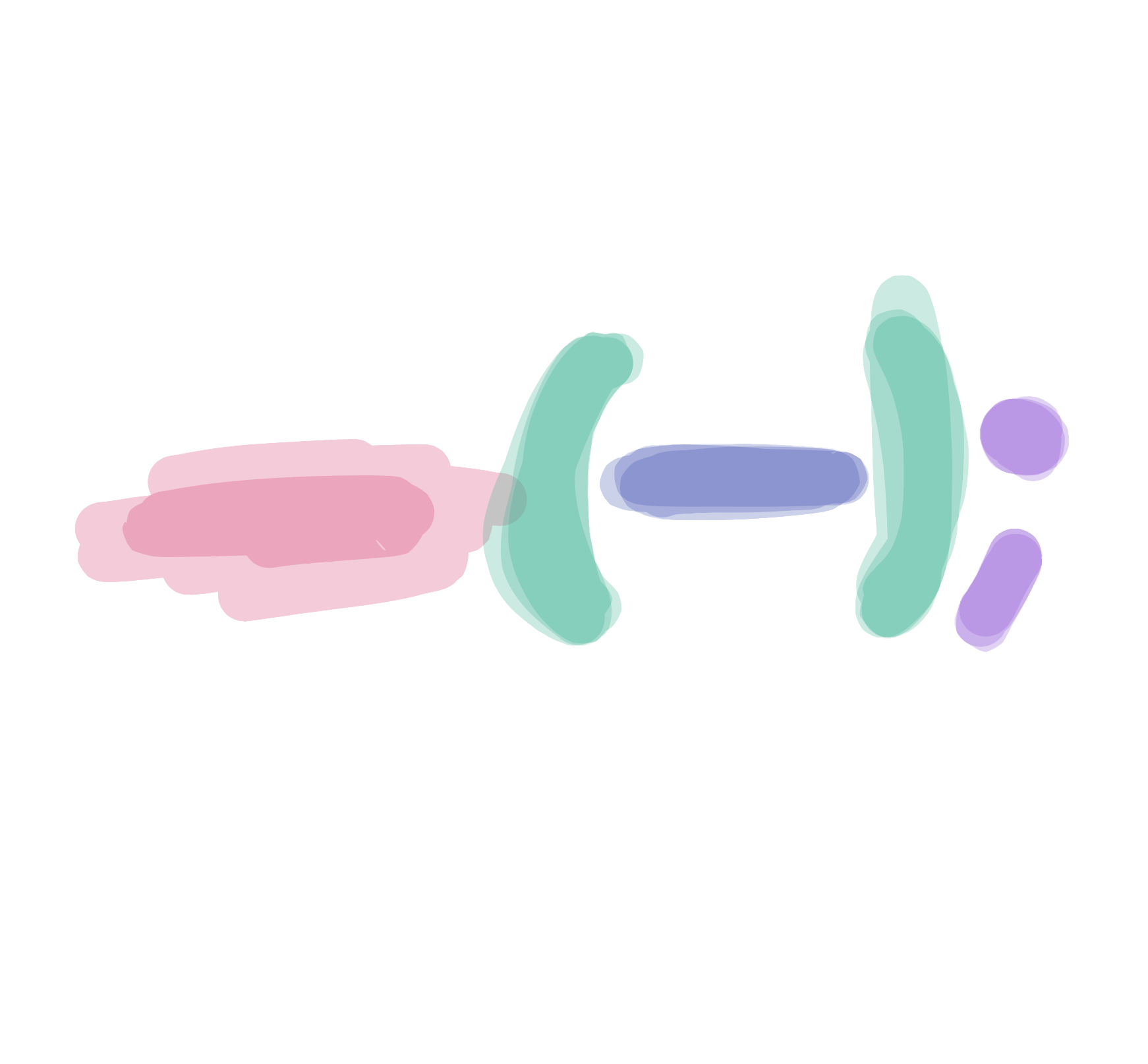
Lesson 5
Drawing more shapes
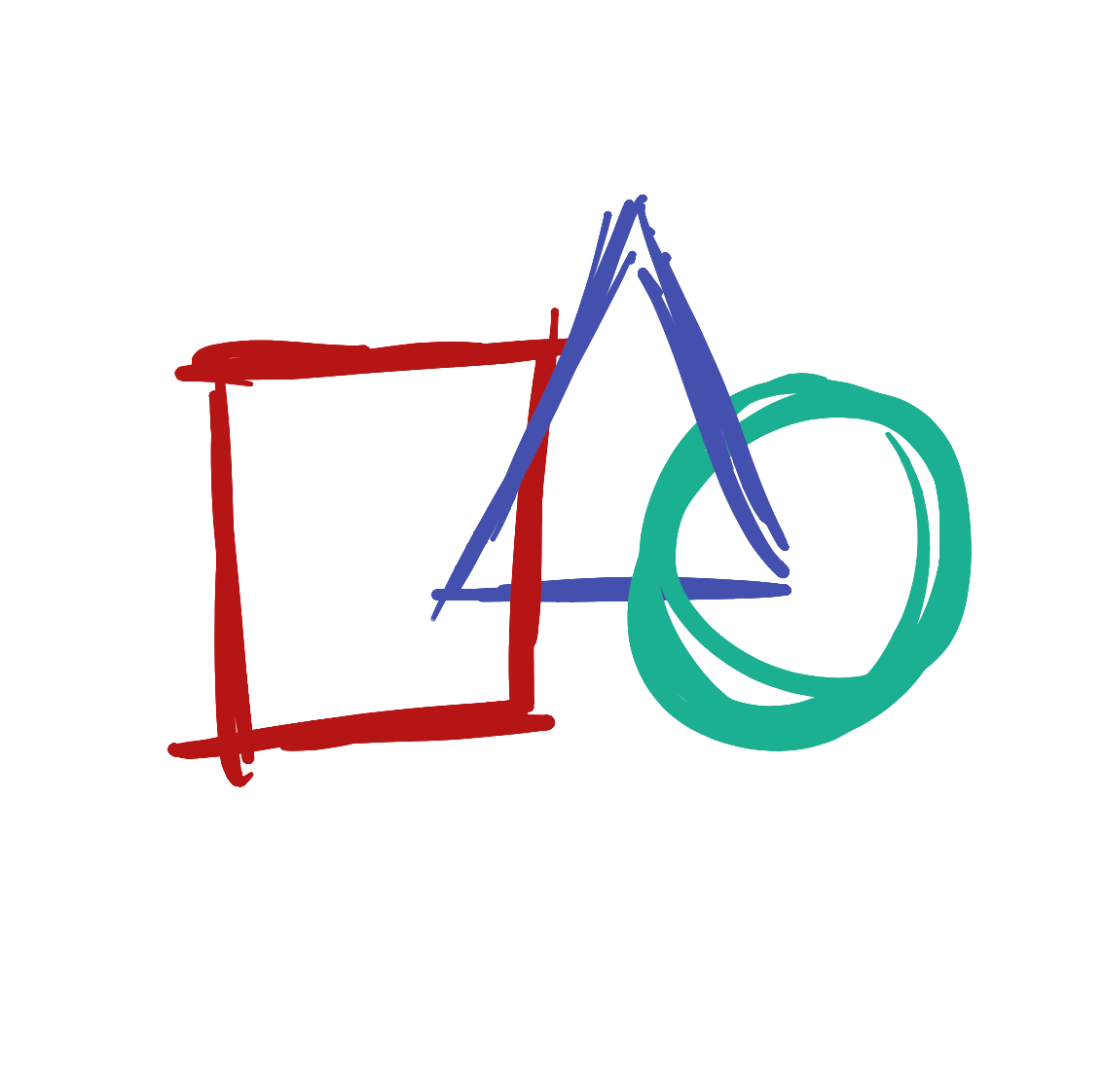
Lesson 6
Placing things on the screen
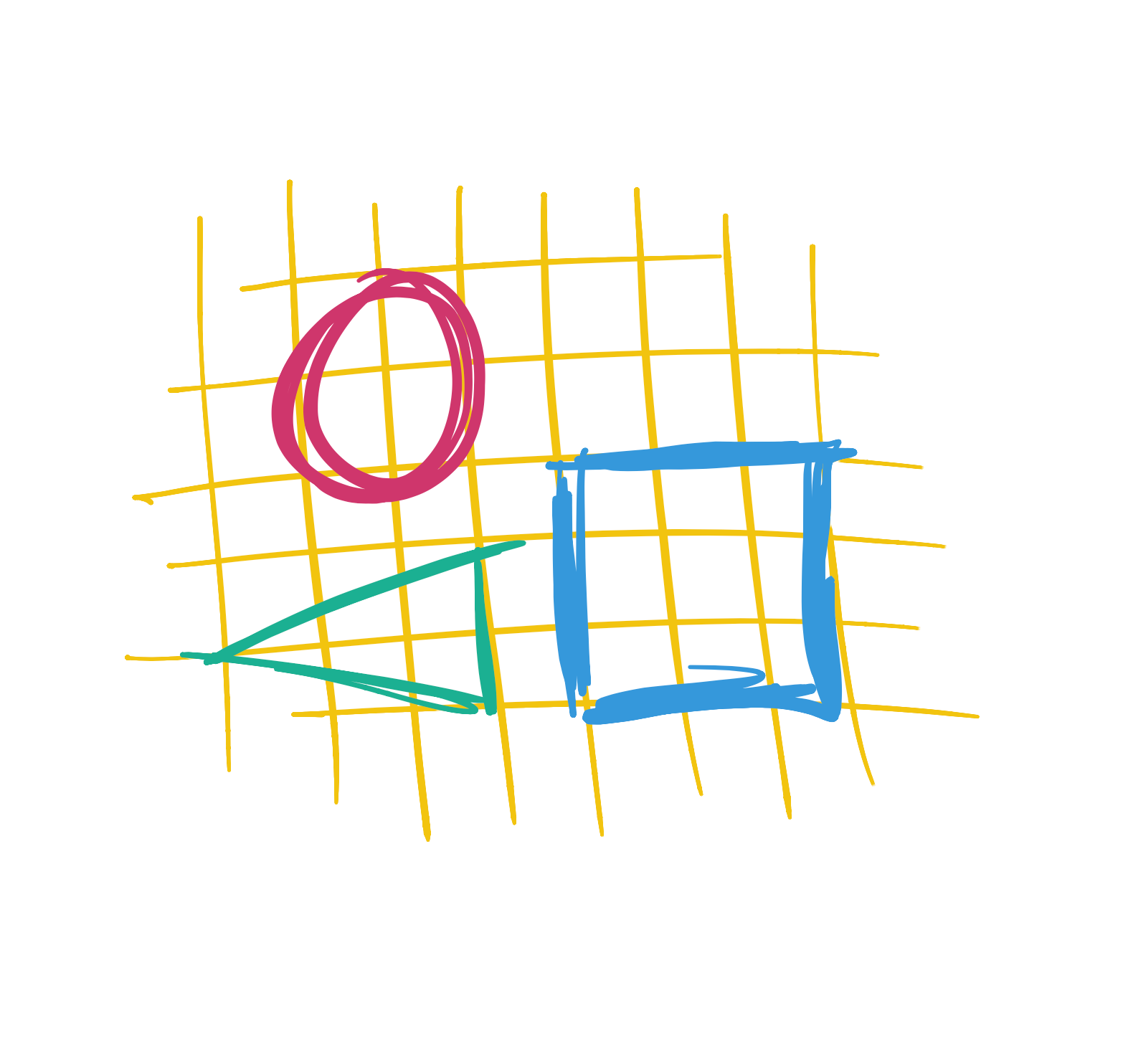
Lesson 7
Black, White, and In Between
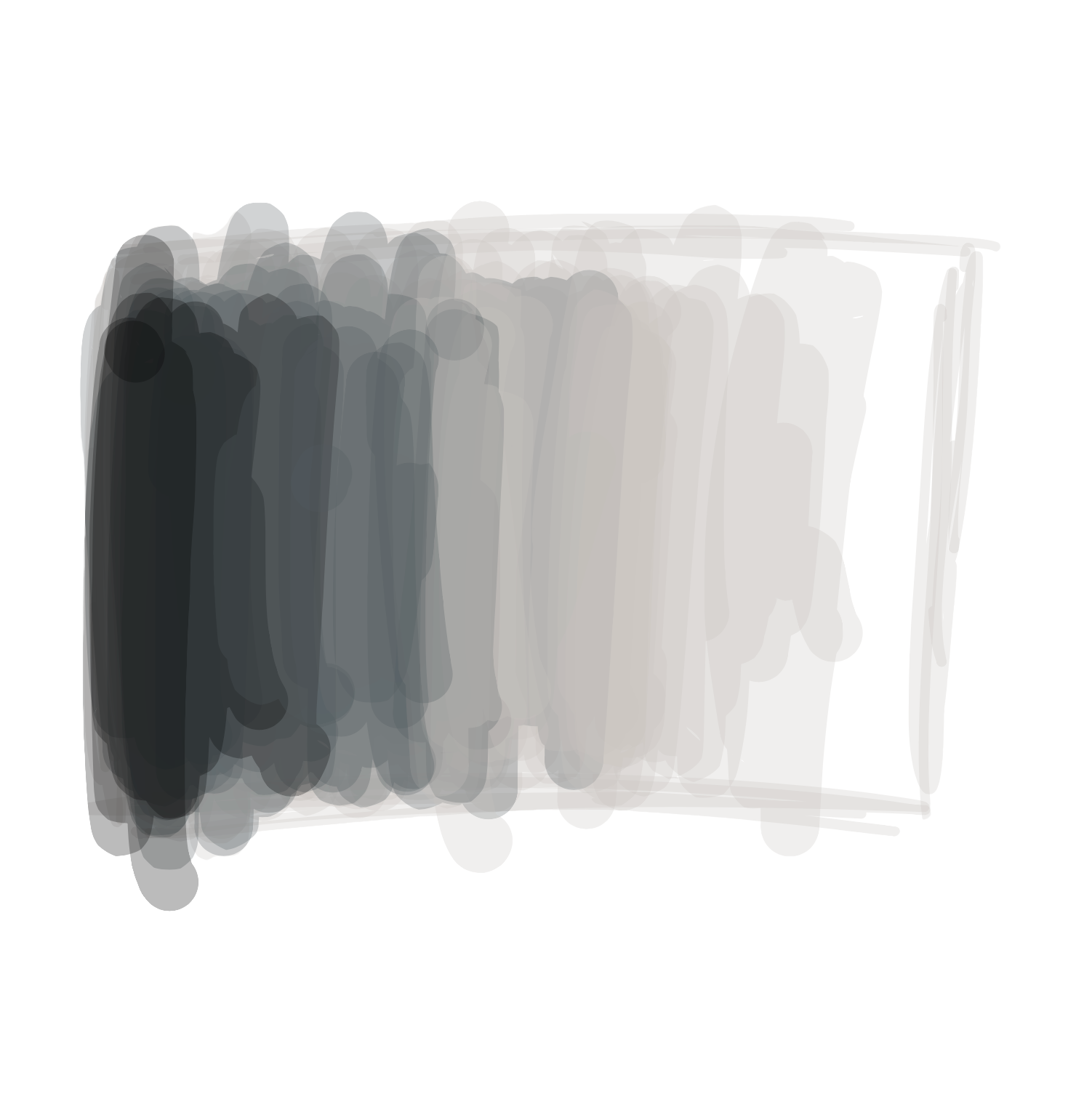
Lesson 8
Color
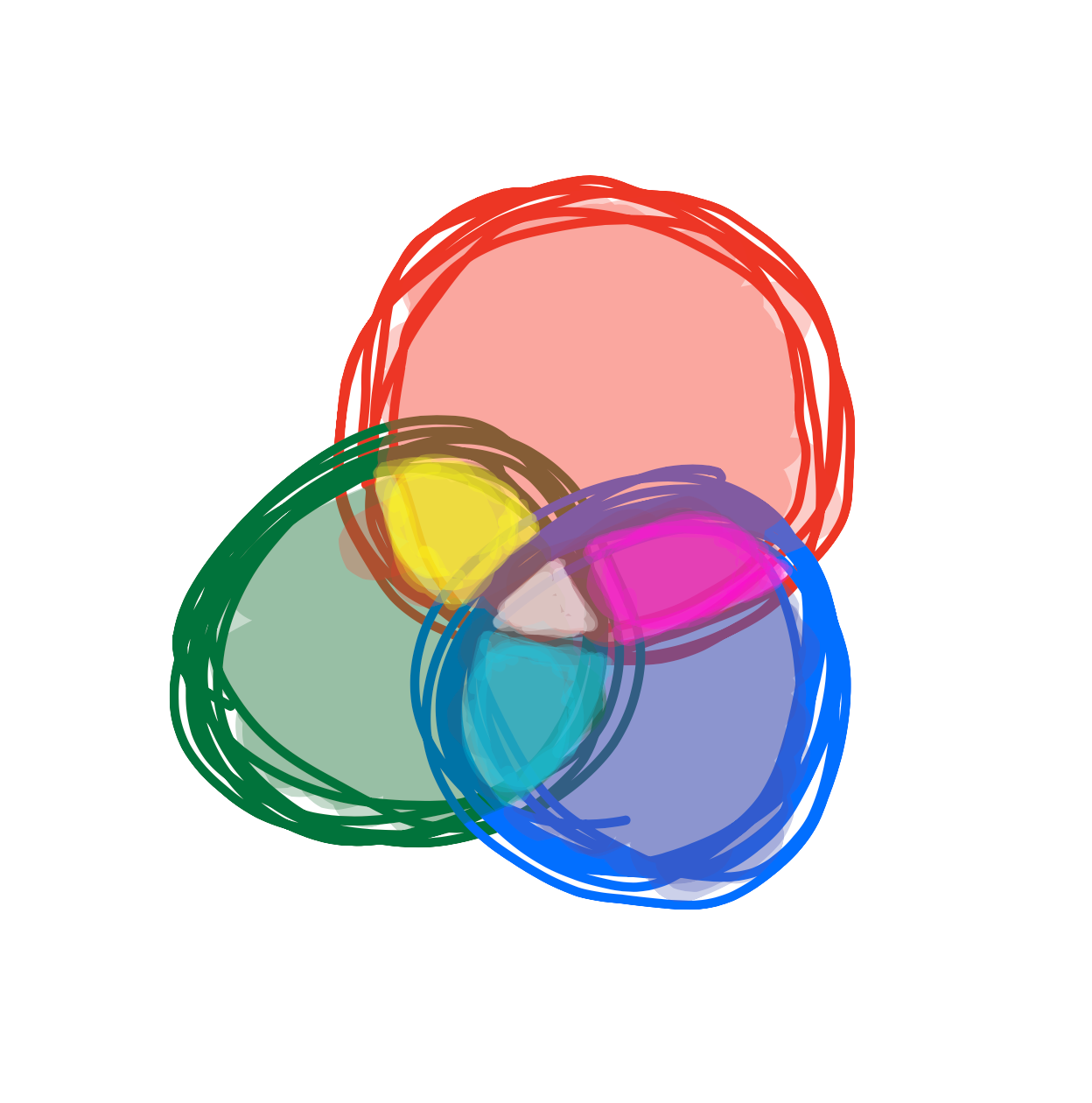
Lesson 9
Fill, Stroke, and Background
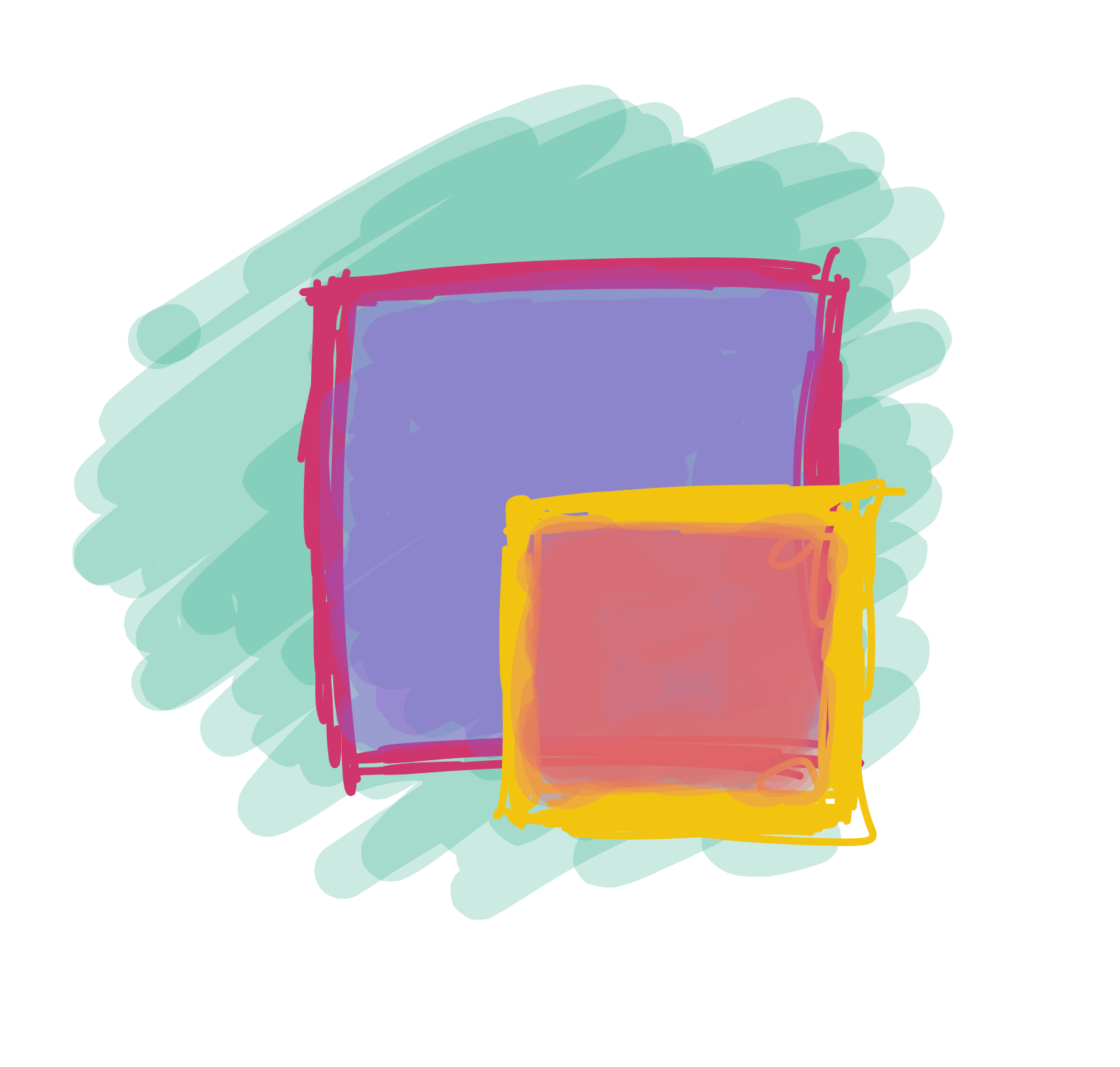
Lesson 10
Variables
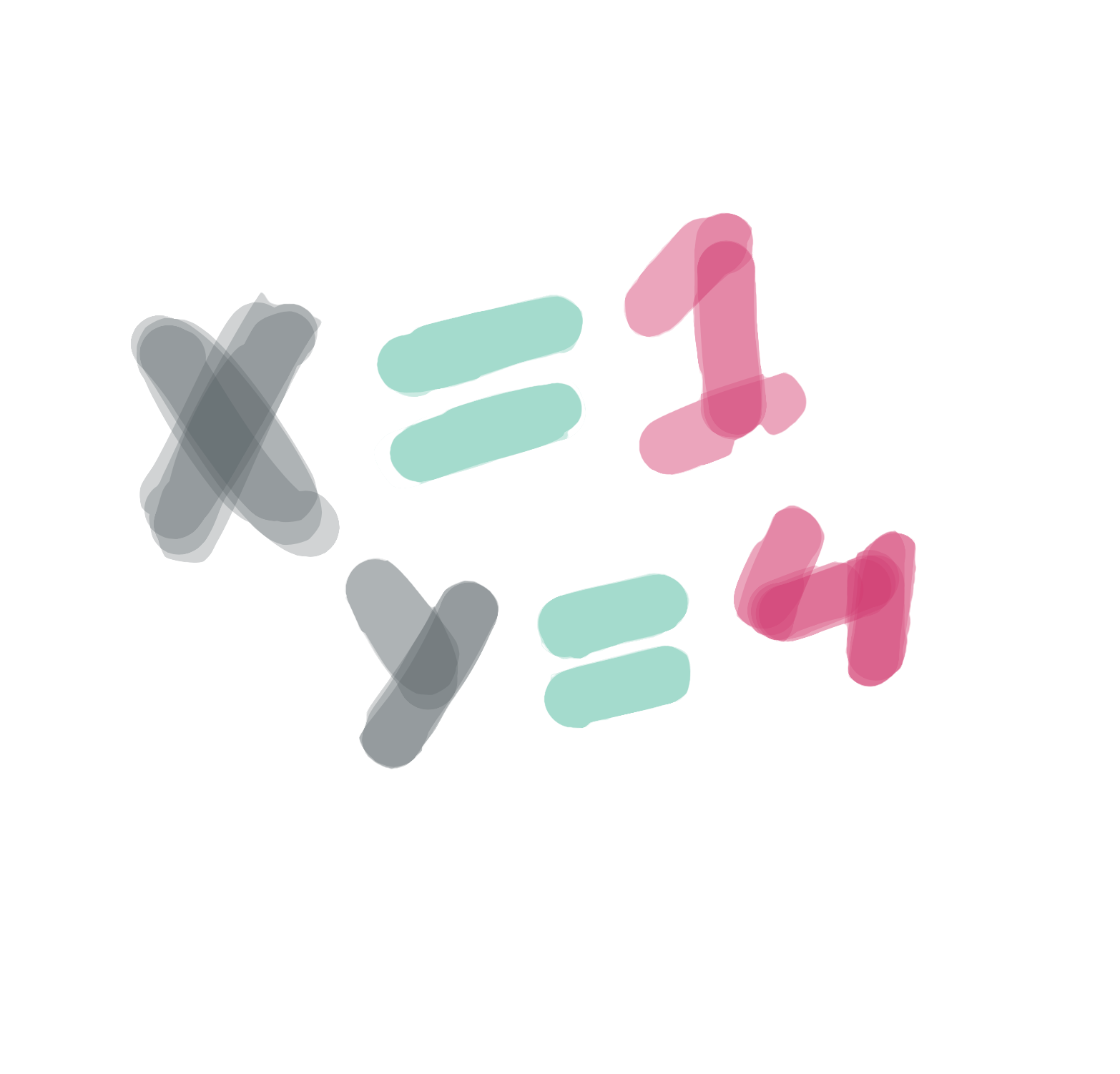
Lesson 11
Making the Screen Bigger
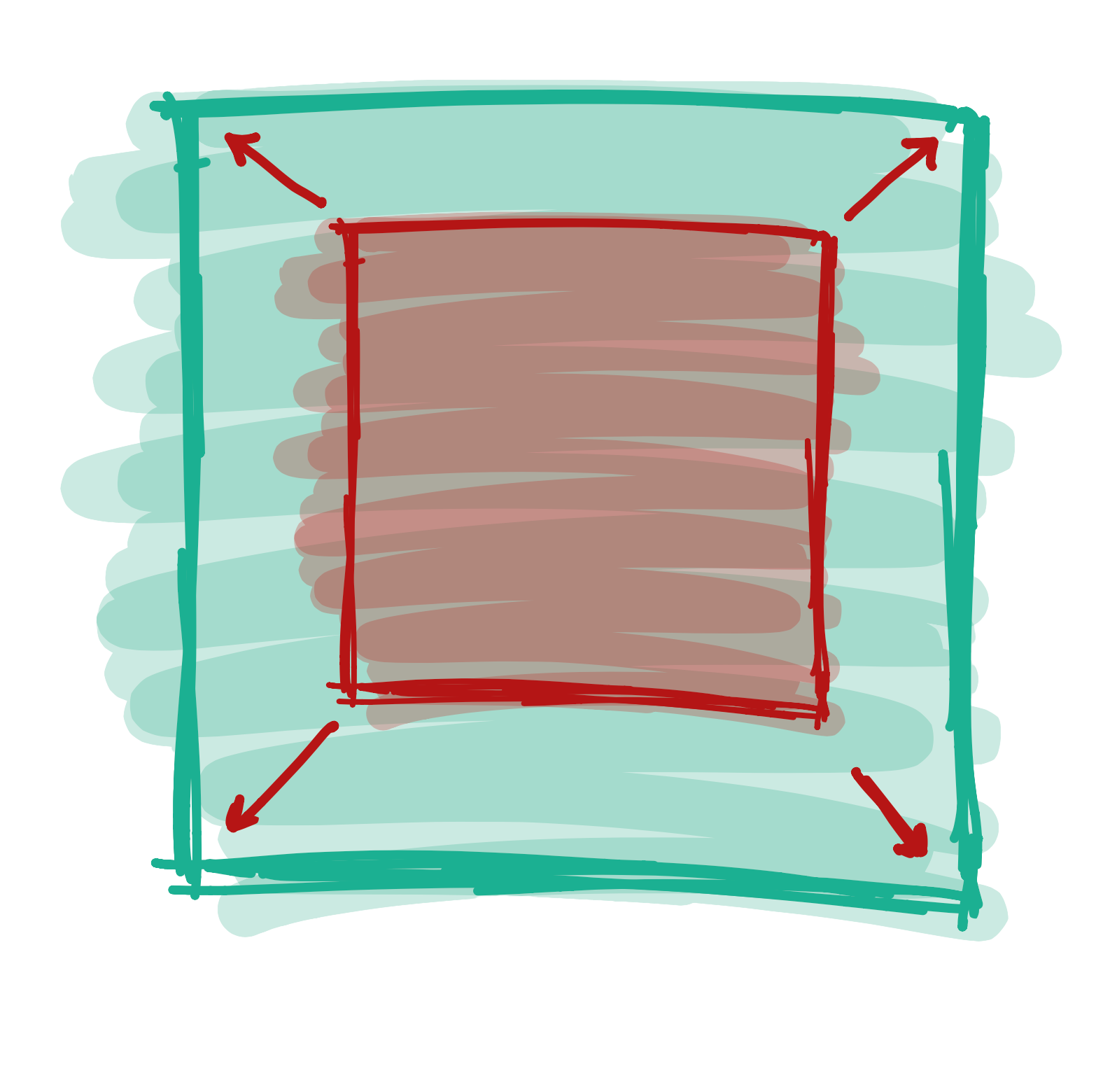
Lesson 12
Modifying Variables
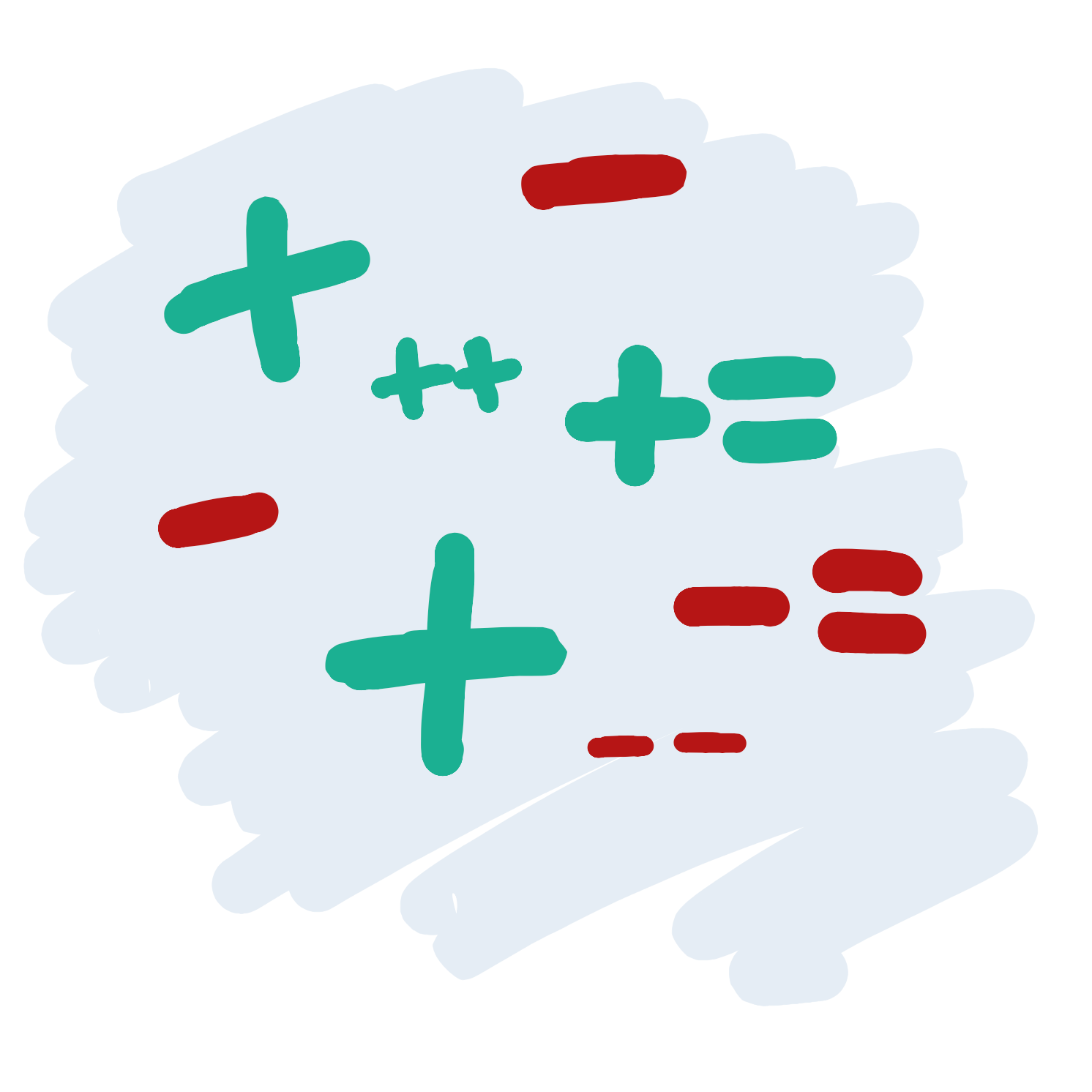
Lesson 13
Functions
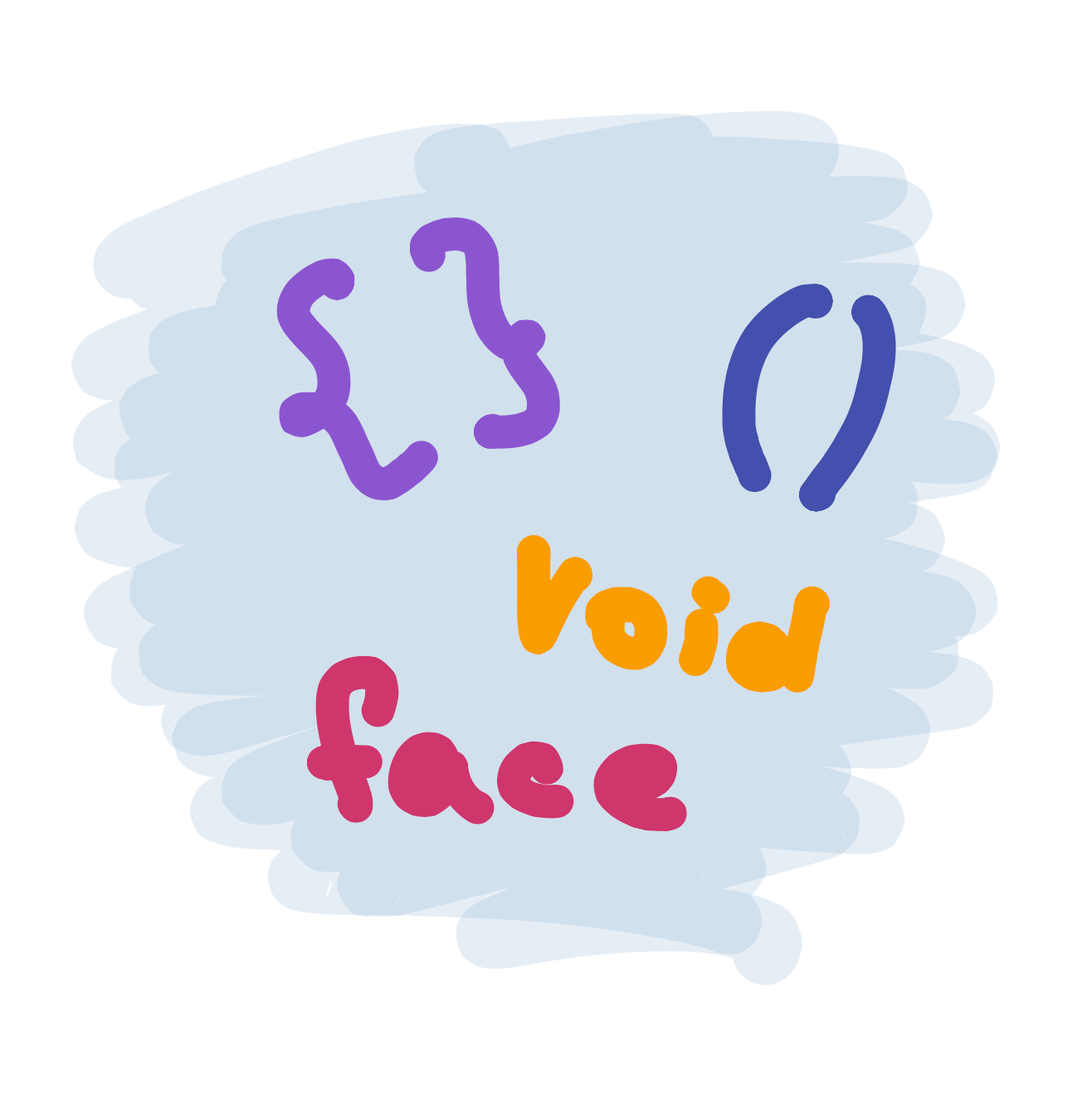
Lesson 14
Animations
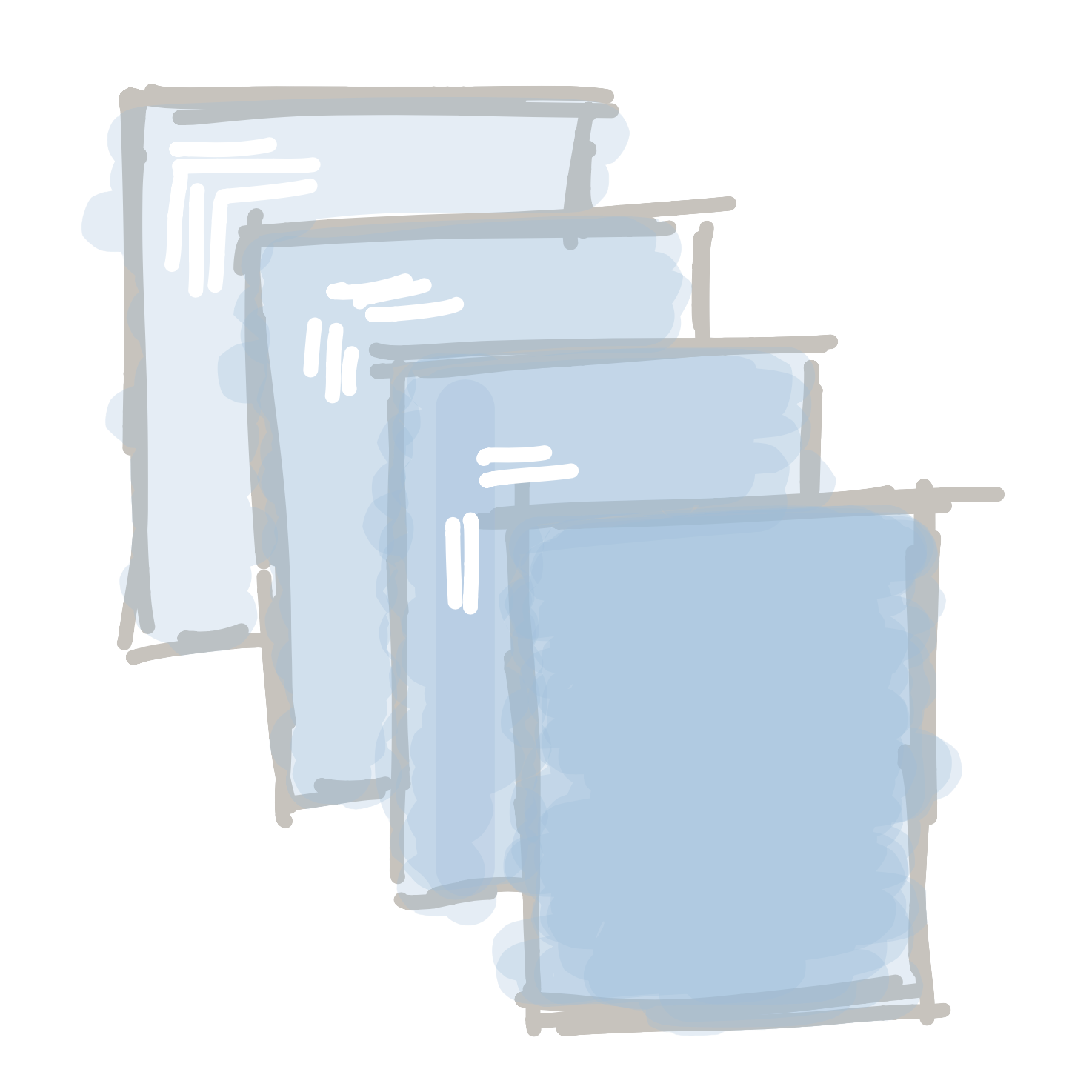
Lesson 15
Let's make a game
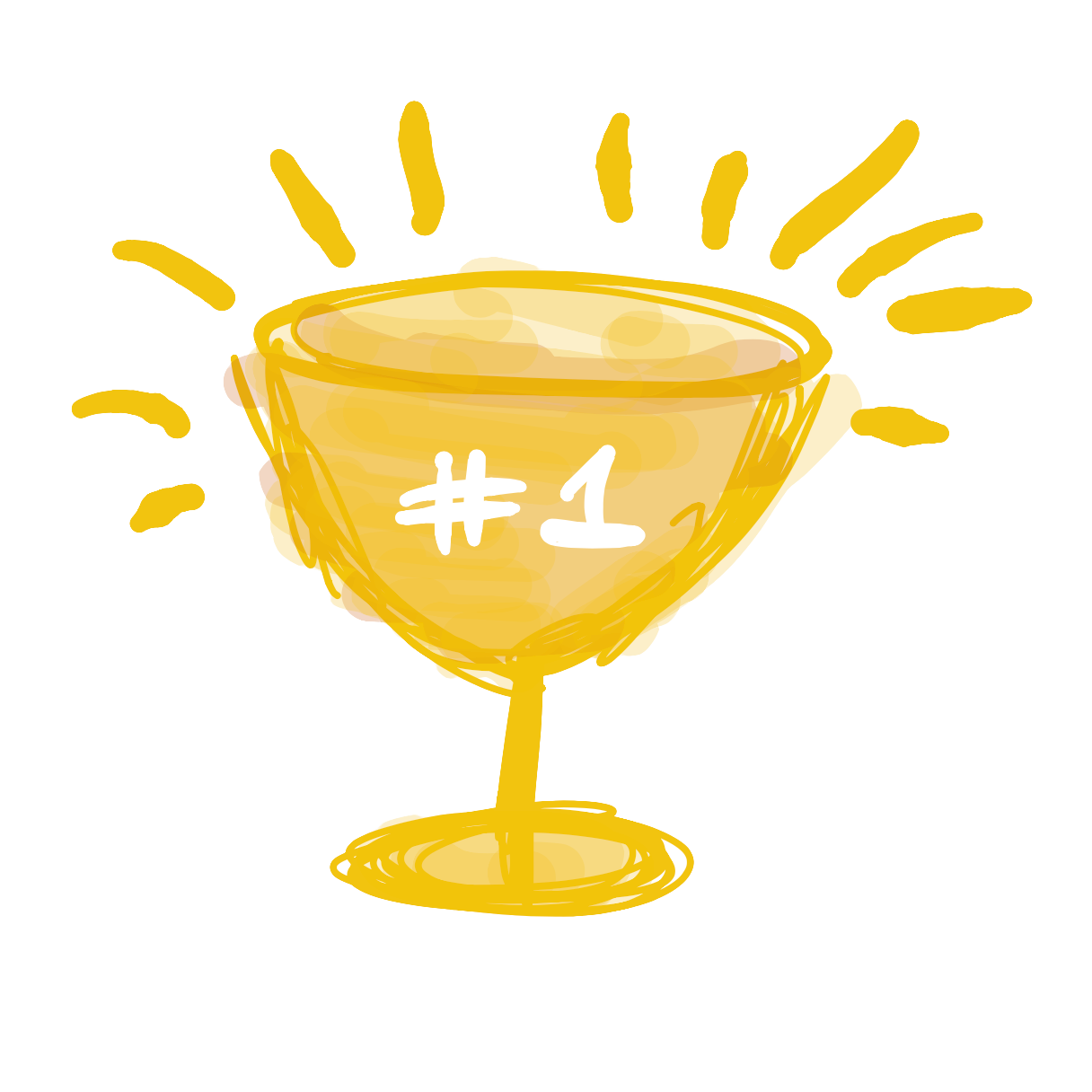
Examples
Use these example Processing programs to learn more about processing
Advanced
Intermediate
Beginner
Reference
Learn more about the functions in Processing
Math
abs() | Calculates the absolute value (magnitude) of a number |
atan2() | Calculates the angle (in radians) from a specified point to the coordinate origin as measured from the positive x-axis |
ceil() | Calculates the closest int value that is greater than or equal to the value of the parameter |
constrain() | Constrains a value to not exceed a maximum and minimum value |
cos() | Calculates the cosine of an angle |
dist() | Calculates the distance between two points |
lerp() | Calculates a number between two numbers at a specific increment |
mag() | Calculates the magnitude (or length) of a vector |
map() | Re-maps a number from one range to another |
max() | Determines the largest value in a sequence of numbers, and then returns that value |
min() | Determines the smallest value in a sequence of numbers, and then returns that value |
noise() | Returns the Perlin noise value at specified coordinates |
norm() | Normalizes a number from another range into a value between 0 and 1 |
pow() | Facilitates exponential expressions |
PVector | A class to describe a two or three dimensional vector, specifically a Euclidean (also known as geometric) vector |
radians() | Converts a degree measurement to its corresponding value in radians |
random() | Generates random numbers |
randomGaussian() | Returns a float from a random series of numbers having a mean of 0 and standard deviation of 1 |
sin() | Calculates the sine of an angle |
sq() | Squares a number (multiplies a number by itself) |
sqrt() | Calculates the square root of a number |
Shape
arc() | Draws an arc to the screen |
beginShape() | Using the beginShape() and endShape() functions allow creating more complex forms |
bezier() | Draws a Bezier curve on the screen |
bezierVertex() | Specifies vertex coordinates for Bezier curves |
ellipse() | Draws an ellipse (oval) to the screen |
ellipseMode() | Modifies the location from which ellipses are drawn by changing the way in which parameters given to ellipse() are intepreted |
endShape() | The endShape() function is the companion to beginShape() and may only be called after beginShape() |
line() | Draws a line (a direct path between two points) to the screen |
point() | Draws a point, a coordinate in space at the dimension of one pixel |
PShape | Datatype for storing shapes |
quad() | A quad is a quadrilateral, a four sided polygon |
rect() | Draws a rectangle to the screen |
rectMode() | Modifies the location from which rectangles are drawn by changing the way in which parameters given to rect() are intepreted |
strokeCap() | Sets the style for rendering line endings |
strokeWeight() | Sets the width of the stroke used for lines, points, and the border around shapes |
triangle() | A triangle is a plane created by connecting three points |
vertex() | All shapes are constructed by connecting a series of vertices |
Color
background() | The background() function sets the color used for the background of the Processing window |
color() | Creates colors for storing in variables of the color datatype |
colorMode() | Changes the way Processing interprets color data |
fill() | Sets the color used to fill shapes |
lerpColor() | Calculates a color between two colors at a specific increment |
noFill() | Disables filling geometry |
noStroke() | Disables drawing the stroke (outline) |
stroke() | Sets the color used to draw lines and borders around shapes |
Data
byte | Datatype for bytes, 8 bits of information storing numerical values from 127 to -128 |
float | Data type for floating-point numbers, e |
int | Datatype for integers, numbers without a decimal point |
Image
copy() | Copies a region of pixels from the display window to another area of the display window and copies a region of pixels from an image used as the srcImg parameter into the display window |
createImage() | Creates a new PImage (the datatype for storing images) |
get() | Reads the color of any pixel or grabs a section of an image |
image() | The image() function draws an image to the display window |
loadPixels() | Loads a snapshot of the current display window into the pixels[] array |
set() | Changes the color of any pixel, or writes an image directly to the display window |
updatePixels() | Updates the display window with the data in the pixels[] array |
Rendering
createGraphics() | Creates and returns a new PGraphics object |
Structure
. (dot) | Provides access to an object's methods and data |
draw() | Called directly after setup(), the draw() function continuously executes the lines of code contained inside its block until the program is stopped or noLoop() is called |
loop() | By default, Processing loops through draw() continuously, executing the code within it |
noLoop() | Stops Processing from continuously executing the code within draw() |
redraw() | Executes the code within draw() one time |
setup() | The setup() function is run once, when the program starts |
super | Keyword used to reference the superclass of a subclass |
Control
for | Controls a sequence of repetitions |
if | Allows the program to make a decision about which code to execute |
Environment
frameRate | The system variable frameRate contains the approximate frame rate of a running sketch |
noSmooth() | Draws all geometry and fonts with jagged (aliased) edges and images when hard edges between the pixels when enlarged rather than interpoloating pixels |
size() | Defines the dimension of the display window width and height in units of pixels |
Input
hour() | Processing communicates with the clock on your computer |
keyPressed() | The keyPressed() function is called once every time a key is pressed |
keyTyped() | The keyTyped() function is called once every time a key is pressed, but action keys such as Ctrl, Shift, and Alt are ignored |
millis() | Returns the number of milliseconds (thousandths of a second) since starting the program |
minute() | Processing communicates with the clock on your computer |
mouseDragged() | The mouseDragged() function is called once every time the mouse moves while a mouse button is pressed |
mousePressed() | The mousePressed() function is called once after every time a mouse button is pressed |
mouseReleased() | The mouseReleased() function is called every time a mouse button is released |
mouseX | The system variable mouseX always contains the current horizontal coordinate of the mouse |
mouseY | The system variable mouseY always contains the current vertical coordinate of the mouse |
second() | Processing communicates with the clock on your computer |
Transform
popMatrix() | Pops the current transformation matrix off the matrix stack |
pushMatrix() | Pushes the current transformation matrix onto the matrix stack |
rotate() | Rotates the amount specified by the angle parameter |
scale() | Increases or decreases the size of a shape by expanding and contracting vertices |
translate() | Specifies an amount to displace objects within the display window |
Output
println() | The println() function writes to the console area, the black rectangle at the bottom of the Processing environment |
Typography
text() | Draws text to the screen |
textAlign() | Sets the current alignment for drawing text |
textAscent() | Returns ascent of the current font at its current size |
textDescent() | Returns descent of the current font at its current size |
textFont() | Sets the current font that will be drawn with the text() function |
textSize() | Sets the current font size |
textWidth() | Calculates and returns the width of any character or text string |
Books
Download a PDF copy of the lessons